AutoRes.uk 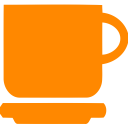
Annotation driven Java™ open source library for automating embedded resource file handling - a convenient alternative to Class.getResourceAsStream.
- Validate resource names with the compiler
- Replace manual resource file I/O handling with generated byte arrays or strings
- Replace localization resource bundle messages with typed method signatures
- Create GraalVM native images without the need for resource manifests
- Extend with additional generators or validators
AutoRes.uk is a compile-time only dependency and does not need to be distributed with application code.
Usage 🔗
Annotate a type or package and specify the resource files.
The processing
library must be exposed to the compiler's annotation processor.
With the
javac
compiler this can be done with the -processorpath
flag.
Sample Code 🔗
@Texts
generates a class that provides text file content as String
s.
@uk.autores.Texts(
name = "Rhymes",
value = {"Poule.txt", "Roses.txt"}
)
public class PrintRhymes {
public static void main(String...args) {
System.out.println(Rhymes.poule()
);
System.out.println(Rhymes.roses()
);
}
}
@Messages
generates typed methods from properties files that format localized strings.
@uk.autores.Messages("msgs.properties")
public class PrintMessage {
public static void main(String[] args) {
var locale = java.util.Locale.getDefault();
int planet = 4;
var time = java.time.ZonedDateTime.now();
var event = "a meteor strike";
// planet-event=At {1,dtf-time} on {1,dtf-date}, there was {2} on planet {0,number,integer}.
// ...becomes signature...
// static String planetEvent(Locale l, Number arg0, ZonedDateTime arg1, CharSequence arg2)
String msg = Msgs.planetEvent(locale, planet, time, event)
;
System.out.println(msg);
}
}
Other annotations are
@ByteArrays
,
@Keys
,
@InputStreams
,
and the extensible @ResourceFiles
.
Tutorials 🔗
- How to Set Up, Compile and Run an AutoRes Project
- How to Verify Java Resource File Paths with the Compiler
- How to Improve Localized String Formatting in Java with Code Generation
Binaries 🔗
Browse the central repository for build system import snippets for the uk.autores:annotations and uk.autores:processing libraries.
Versioning & Compatibility 🔗
Versions are split into three integers: Java version; major version; minor version. Browse the central repository to see available versions.
Functionally identical Java 8 and Java 11 versions are published. The Java 11 version has marginally better encapsulation when modules are in use.
Java Module System 🔗
Use requires static uk.autores
in
module-info.java to depend on this library at compile time only.
module example.module.name {
requires static uk.autores;
}
Apache Maven 🔗
Apache Maven projects should use the provided scope.
<!-- Maven 3 -->
<dependency>
<groupId>uk.autores</groupId>
<artifactId>annotations</artifactId>
<version>${autores.version}</version>
<scope>provided</scope>
</dependency>
Make the compiler aware of the annotation processor using annotationProcessorPaths.
<!-- Maven 3 -->
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>${maven.compiler.plugin.version}</version>
<configuration>
<annotationProcessorPaths>
<path>
<groupId>uk.autores</groupId>
<artifactId>processing</artifactId>
<version>${autores.version}</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
</plugins>
</build>
Gradle 🔗
Gradle projects must add resource files to the classpath. The processing library must be exposed to the annotation processor.
// Gradle 8; Kotlin syntax
dependencies {
compileOnly(files("src/main/resources"))
compileOnly("uk.autores:annotations:" + autoresVersion)
annotationProcessor("uk.autores:processing:" + autoresVersion)
}